React Native Social Authentication Using Firebase: Google+ and Facebook Signin, Signup – A Step by Step Guide
One has to go through following steps to enable social authentication in their react native mobile apps. In this tutorial we will see how to enable react native social login for android applications.
React Native Google Plus Signup/Signin Using Firebase Authentication
1. Create Project in Google Cloud Platform : Try free trail
2. Enable Google+ API for your project and generate API Key
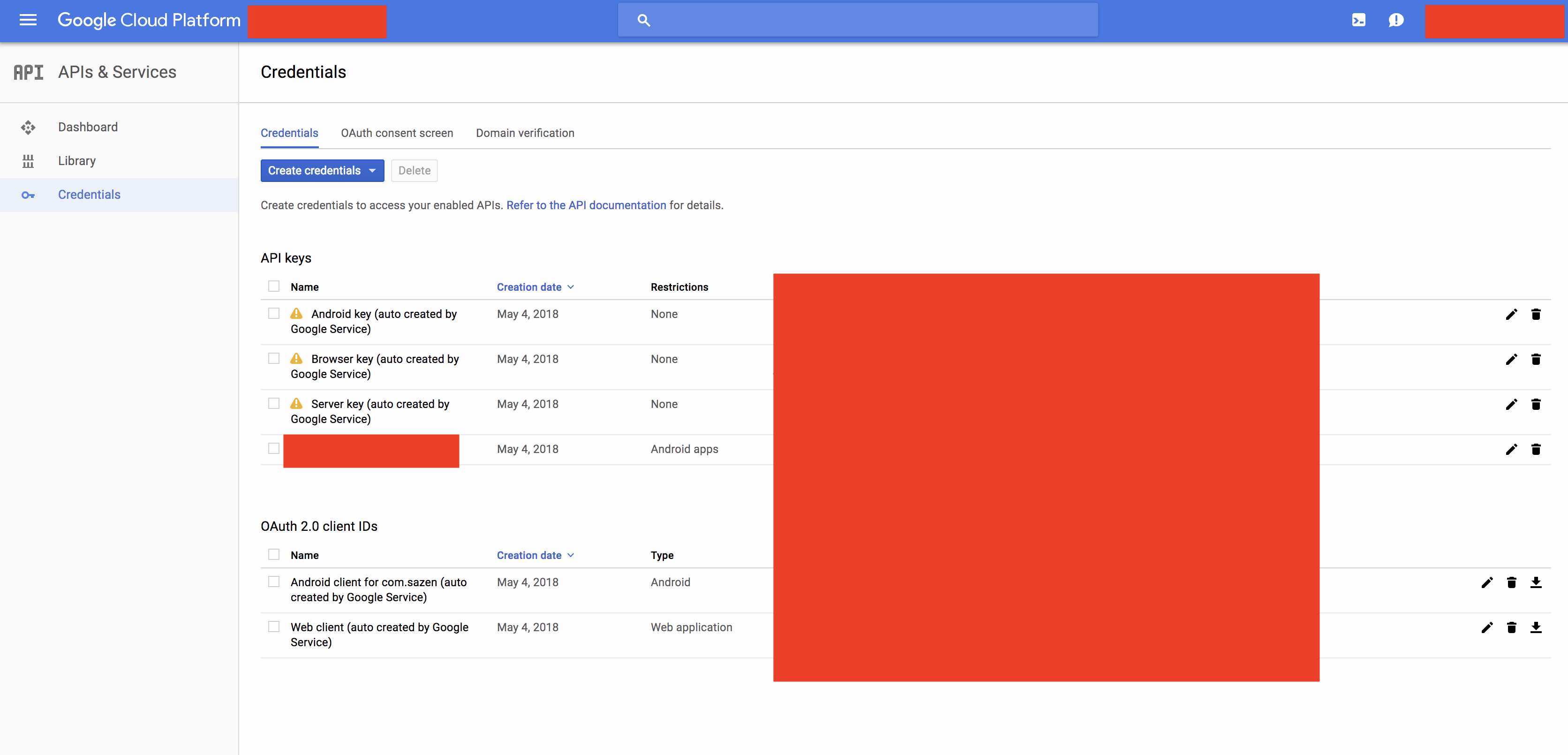
3. Set YourSHA-1 key and package name (as given in AndroidManifest.xml ) of your android project
To generate your own SHA-1 use the command -> keytool -list -v -keystore mystore.keystore
NOTE : If Your build is debug build then theSHA-1 generated with above command won’t work, kindly check your adb logs carefully, theSHA-1 being used by your android debug build will be logged in the device log. To check device log run the below command from your /Users/<YourSystemUserName>/Library/Android/sdk/platform-tools —> adb logcat

4. Import the same project in firebase
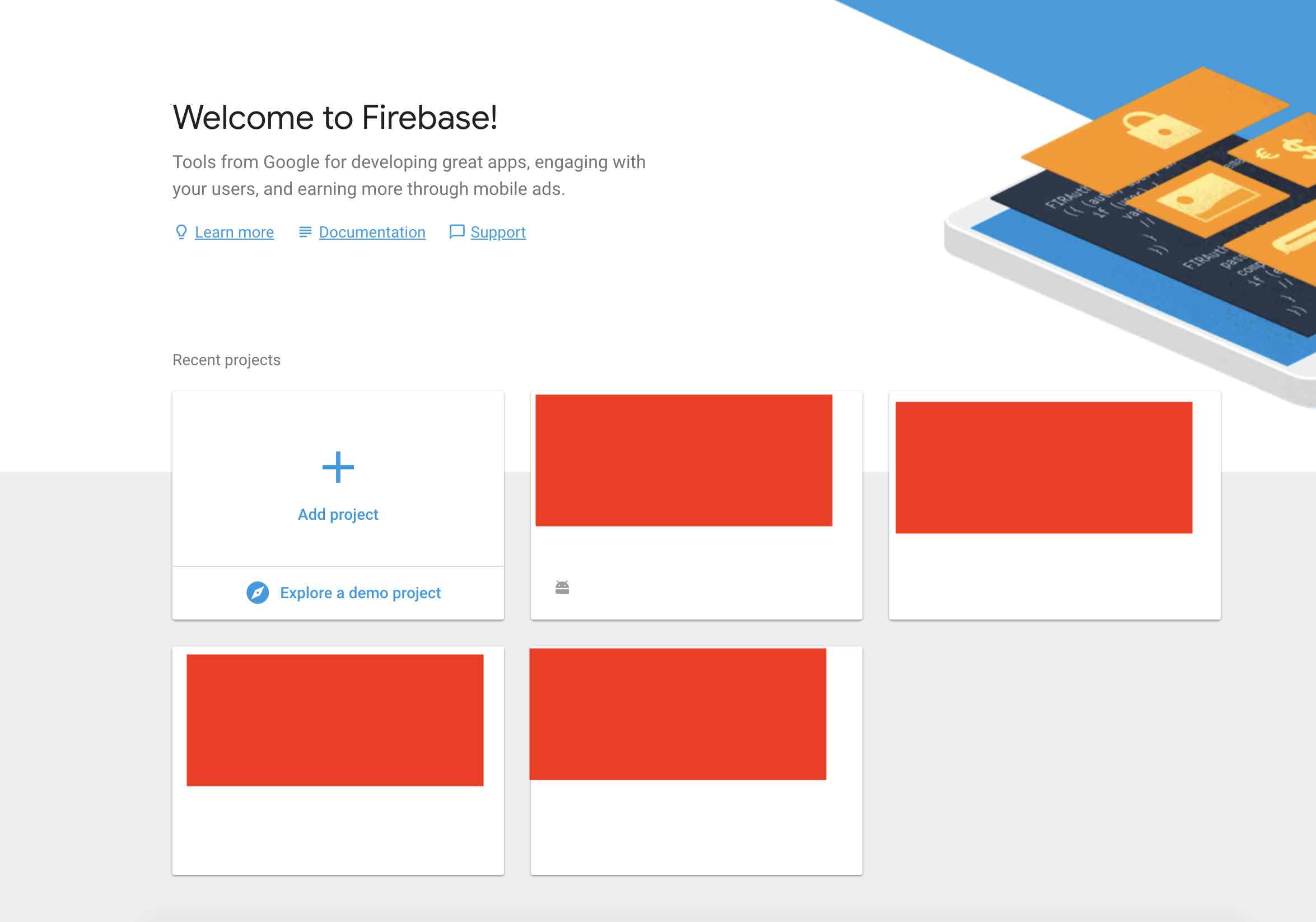
5. Setup android project in your firebase project, then setup authentication methods being used in your app.

6. Then setup sameSHA-1 Key for your firebase project: Navigate to Project setting from side bar and click on general , select android app and set SHA-1 key
NOTE: Once SHA-1 is setup, download google-services.json file in the same page. and keep the file under your android project director app folder /ReactNativeProjectFolder/android/app
Till this your google cloud and firebase setup is done, now we have to do lot of changes in code.
7. add ‘react-native-google-signin’ and ‘firebase’ modules
npm install react-native-google-signin –save
npm install firebase –save
8. Add dependency in your /app/bundle.gradle
Note : in below code those excludes are most important, or else you will encounter strange linking errors.
implementation project(':react-native-google-signin')
if your app is using some other dependencies like react-native-maps or react-native-social-share then do below changes as well
implementation(project(":react-native-google-signin")){ exclude group: "com.google.android.gms" // very important } compile(project(':react-native-maps')) { exclude group: 'com.google.android.gms', module: 'play-services-base' exclude group: 'com.google.android.gms', module: 'play-services-maps' } implementation 'com.google.android.gms:play-services-base:11.+' implementation 'com.google.android.gms:play-services-maps:11.+'
9. your android/bundle.gradle file should look as follows
// Top-level build file where you can add configuration options common to all sub-projects/modules. buildscript { repositories { google() jcenter() } dependencies { classpath 'com.android.tools.build:gradle:3.0.1' classpath 'com.google.gms:google-services:3.0.0' // <--- add this // NOTE: Do not place your application dependencies here; they belong // in the individual module build.gradle files } } allprojects { repositories { mavenLocal() jcenter() maven { // All of React Native (JS, Obj-C sources, Android binaries) is installed from npm url "$rootDir/../node_modules/react-native/android" } } } ext { compileSdkVersion = 23 targetSdkVersion = 23 buildToolsVersion = "23.0.1" googlePlayServicesVersion = "10.2.4" androidMapsUtilsVersion = "0.5+" }
10. run below commands
npm install
react-native link
11. Once you run react native link – check android/settings.gradle file , cross check that there should not be duplicate lines of content.
So far we have done project level configurations, now let us see code changes
12. React Native Google sign-in/sign-up using firebase code
import { GoogleSignin } from 'react-native-google-signin'; import firebase from 'firebase'; function googleAuthConfig(successCallback, failureCallback) { /* Web Client id Get the client_id from Google-services.json file, in that file under "client": [ "oauth_client": [ { "client_id": "", //This you have to use as webClientId and the same for android "client_type": 3 } */ GoogleSignin.configure({ iosClientId: CLIENT_IDS.GOOGLE_IOS_CLIENT_ID, webClientId: '', hostedDomain: '', // specifies a hosted domain restriction forceConsentPrompt: true, // [Android] if you want to show the authorization prompt at each login accountName: '' // [Android] specifies an account name on the device that should be used }) .then(() => { console.log('Google Config Success'); successCallback(); }) .catch((err) => { console.log('Google Config Error'); failureCallback(err); }); } function googleSignin() { googleAuthConfig(() => { GoogleSignin.signIn() .then((user) => { const { accessToken } = user; var credentials = firebase.auth.GoogleAuthProvider.credential(null, accessToken); firebase.auth().signInWithCredential(credentials) .then((firebaseResult) => { loginToSG(dispatch, firebaseResult, props, 'Google', registerCallback); }) .catch(error => console.log('error while checking with firebase', error)); }) .catch((err) => { console.log(err); }); }, (googleConfigErr) => { console.log(googleConfigErr); }); }
13. Finally the most important step is -> once do npm cache clean, delete your existing app from device, delete build folders then run the app
react-native run-android